How To Create Maps With React And Leaflet

- Published on
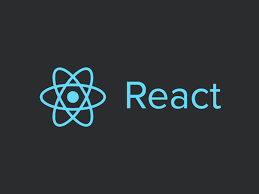
Sure, here is the blog post in Markdown:
Creating Maps With React And Leaflet
Introduction
Incorporating interactive maps into web applications is an effective way to visualize and explore geographical data. By leveraging the power of React and Leaflet, you can seamlessly integrate dynamic maps into your web development projects. This guide will walk you through the process of creating maps with React and Leaflet, equipping you with the essential knowledge to enhance your web applications with compelling visual representations of geographical data.
Prerequisites
Before embarking on this journey, ensure you have the following prerequisites:
- Basic understanding of React and JavaScript
- Node.js and npm installed on your system
Setting up the React App
- Create a React project using Create React App:
npx create-react-app react-leaflet-map
- Navigate into the project directory:
cd react-leaflet-map
Installing Leaflet and React Leaflet
- Install the Leaflet library and its React wrapper,
react-leaflet
:
npm install leaflet react-leaflet
Creating a Basic Map
Create a new component file,
Map.js
, to render the map.Import the
Map
,TileLayer
, andMarker
components fromreact-leaflet
:
import React from 'react';
import { Map, TileLayer, Marker } from 'react-leaflet';
- Define the map coordinates:
const mapCenter = [51.505, -0.09];
- Define the zoom level:
const mapZoom = 13;
- Create the React component for the map:
const MapComponent = () => {
return (
<Map
center={mapCenter}
zoom={mapZoom}
style={{ width: '100%', height: '400px' }}
>
<TileLayer
url="https://{s}.tile.openstreetmap.org/{z}/{x}/{y}.png"
attribution='© <a href="https://www.openstreetmap.org/copyright">OpenStreetMap contributors</a>'
/>
<Marker position={mapCenter}>
<Popup>I'm here!</Popup>
</Marker>
</Map>
);
};
Displaying the Map
- Import and use the
MapComponent
in your main component, such asApp.js
:
import React from 'react';
import MapComponent from './MapComponent';
const App = () => {
return (
<div className="App">
<MapComponent />
</div>
);
};
export default App;
- Run the React app:
npm start
Enhancing the Map
Add more markers to represent different locations.
Use custom icons for markers to make them more visually appealing.
Implement interactive features, such as popups on click or drag-and-drop markers.
Explore advanced Leaflet features, such as polylines, polygons, and heatmaps, to visualize complex data.
Conclusion
Creating maps with React and Leaflet empowers you to build interactive and informative data visualizations. Start with the basics, and gradually incorporate more complex features to create sophisticated maps that enhance your web applications.