How To Create A Card Matching Game Using React And RxJS

- Published on
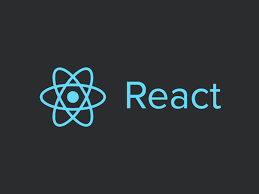
🃏 How To Create A Card Matching Game Using React And RxJS 🚀
Hey Laraib Senpai! Ready to embark on a thrilling journey of creating a card matching game with the dynamic duo of React and RxJS? Let's dive in!
1. Set Up Your React Project 🌐
Start by creating a new React project using Create React App or your preferred setup. Ensure you have Node.js and npm installed.
npx create-react-app card-matching-game
cd card-matching-game
2. Install RxJS 🔄
Install RxJS to leverage its powerful reactive programming capabilities.
npm install rxjs
3. Create Card Components 🃏
Build React components for your cards. Each card will have a state to track whether it's flipped or matched.
// Card.js
import React from 'react';
const Card = ({ id, content, isFlipped, isMatched, onCardClick }) => (
<div
className={`card ${isFlipped ? 'flipped' : ''} ${isMatched ? 'matched' : ''}`}
onClick={() => onCardClick(id)}
>
{isFlipped || isMatched ? content : '🎴'}
</div>
);
export default Card;
4. Set Up the Game Board 🎮
Create a component for the game board that renders a grid of cards. Use state to manage the game logic.
// GameBoard.js
import React, { useState, useEffect } from 'react';
import Card from './Card';
const GameBoard = () => {
const [cards, setCards] = useState(/* Initial card data */);
useEffect(() => {
// Initialize and shuffle the cards
// ...
// Subscribe to card clicks using RxJS
// ...
// Clean up subscriptions
return () => {
// Unsubscribe here
};
}, []);
const handleCardClick = (cardId) => {
// Handle card clicks and check for matches
// ...
};
return (
<div className="game-board">
{cards.map((card) => (
<Card
key={card.id}
id={card.id}
content={card.content}
isFlipped={card.isFlipped}
isMatched={card.isMatched}
onCardClick={handleCardClick}
/>
))}
</div>
);
};
export default GameBoard;
5. Style Your Game 🎨
Add some CSS to make your game visually appealing. You can use a simple grid layout for the cards.
/* styles.css */
.card {
/* Card styles */
}
.flipped {
/* Flipped card styles */
}
.matched {
/* Matched card styles */
}
.game-board {
/* Game board styles */
}
6. Run Your React App 🚀
Start your React app and see your card matching game in action!
npm start
7. Enhance with Animations and Effects 🌈
Leverage RxJS for animations and effects, adding a layer of dynamism to your game.
8. Test and Debug ⚙️
Thoroughly test your game, ensuring that card flipping, matching, and game logic work seamlessly.
9. Share Your Creation! 🎉
Share your card matching game on social media or GitHub. Don't forget to showcase your React and RxJS skills!
Feel free to use this Markdown guide as a starting point for your React and RxJS card matching game project!